How To Create an Array of Unique Values in JavaScript
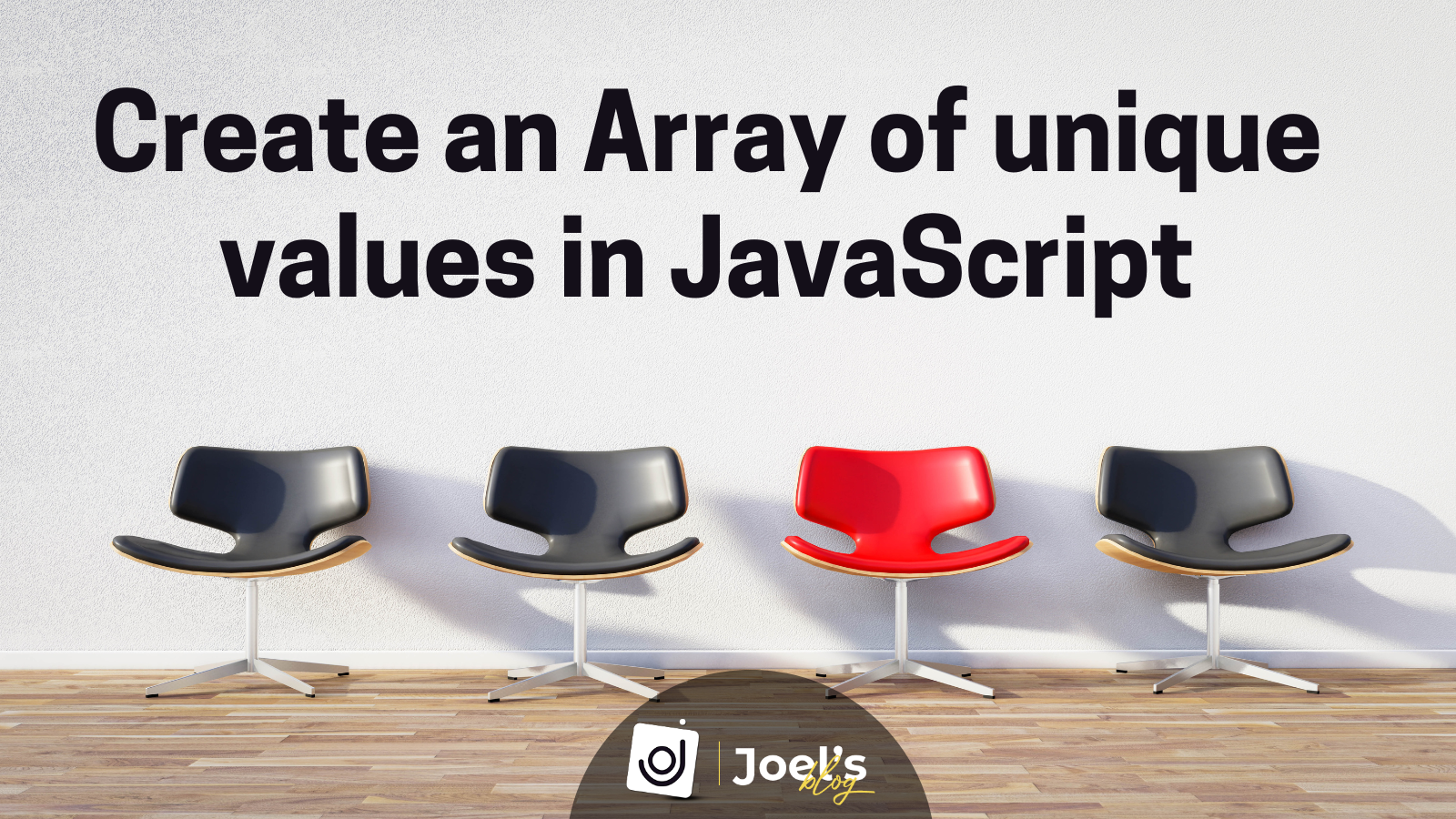
As a programmer, you know that arrays are a powerful tool in JavaScript. However, sometimes you need to ensure that the data in your array is unique.
Why? Imagine if you had a list of names, and some of them were repeated. It would make it difficult to find a specific name, wouldn't it? That's why having an array of unique values is crucial.
In this article, you’ll learn how to create an array of unique values in JavaScript. This article will cover three methods that are easy to implement and will save you time and headaches in the long run.
So let's dive in and get started!
What Is an Array?
Arrays are a powerful feature of JavaScript that allows you to store and manipulate data. An array is simply a list of items, where each item can be accessed using an index number (JavaScript arrays are zero-indexed).
In JavaScript, an array is defined using square brackets, and commas separate items in the array. For example, an array of numbers might look like this:
const numbers = [1, 2, 3, 4, 5];
Arrays can hold any data type, including strings, objects, and other arrays. Using various built-in methods, you can add, remove, or modify items in an array.
Arrays are used in many different ways in JavaScript. For example, they can store user input, manipulate and display data in a web application, or even create dynamic animations. The possibilities are endless!
One of the most powerful aspects of arrays is their ability to be iterated using loops or higher-order functions like map()
or reduce()
. This makes it easy to perform complex operations on large data sets with just a few lines of code.
What Are Unique Values?
Now that we know what arrays are let's talk about unique values. In JavaScript, a unique value is simply a value that appears only once in a given dataset.
For example, let's say we have an array of colors:
const colors = ['red', 'blue', 'green', 'yellow', 'red', 'blue'];
In this case, the values 'red' and 'blue' appear twice, while 'green' and 'yellow' appear only once. To create an array of unique values from this dataset, we would need to remove the duplicate 'red' and 'blue' values.
Arrays with non-unique values can cause programming problems, leading to incorrect calculations or unexpected behavior. That's why it's important to identify and remove duplicate values whenever possible.
Luckily, JavaScript provides several methods for creating an array of unique values. We'll cover three of the most commonly used methods in this article.
Methods To Create an Array of Unique Values in JavaScript
Now that you understand what arrays and unique values are and why they're important, let's explore some methods for creating an array of unique values in JavaScript.
Method 1: Using the Set Object
The Set object is a built-in object in JavaScript that allows you to create a collection of unique values.
Duplicates are automatically removed when you add values to a Set object.
To create an array of unique values using a Set object, we can simply pass the original array into the Set constructor and then convert the Set back into an array using the spread operator. Here's an example:
const originalArray = ['apple', 'banana', 'cherry', 'apple', 'banana'];
const uniqueArray = [...new Set(originalArray)];
console.log(uniqueArray); // returns ['apple', 'banana', 'cherry']
Method 2: Using the filter() and indexOf() Methods
The filter()
method creates a new array with all elements that pass a certain test. Combining it with the indexOf() method can create an array of unique values. The indexOf()
method returns the first index at which a given element can be found in the array.
Here's an example of how to use the filter()
and indexOf()
methods to create an array of unique values:
const originalArray = ['apple', 'banana', 'cherry', 'apple', 'banana'];
const uniqueArray = originalArray.filter((value, index, self) => {
return self.indexOf(value) === index;
});
console.log(uniqueArray); // returns ['apple', 'banana', 'cherry']
Method 3: Using the reduce() and includes() Methods
The reduce()
method reduces an array to a single value by iterating over each element and applying a function. You can use the reduce()
method to create an array of unique values by checking whether the array already includes the current value.
Here's an example of how to use the reduce()
and includes()
methods to create an array of unique values:
const originalArray = ['apple', 'banana', 'cherry', 'apple', 'banana'];
const uniqueArray = originalArray.reduce((accumulator, currentValue) => {
if (!accumulator.includes(currentValue)) {
accumulator.push(currentValue);
}
return accumulator;
}, []);
console.log(uniqueArray); // returns ['apple', 'banana', 'cherry']
These three methods provide different approaches to creating various unique values in JavaScript. Depending on your specific use case, one method may be more appropriate.
Try them out and see which one works best for you!
Conclusion
In this article, you have explored three methods for creating an array of unique values in JavaScript.
The first method involves using the Set object, which automatically removes duplicates when you pass an array into it. The second method uses the filter() and indexOf() methods to create a new array with only the unique values from the original array. Finally, the third method uses the reduce() and includes() methods to create a new array of unique values by checking whether the array already includes the current value.
In programming, unique values are important for various reasons, such as ensuring the accuracy of data and avoiding errors that can occur from duplicates. By using these methods to create an array of unique values in JavaScript, you can improve the performance and reliability of your code.
It's important to note that there are often multiple ways to solve a programming problem, and it's up to you to choose the best method for your specific use case. Experiment with these methods and see which one works best for you.
In summary, creating an array of unique values in JavaScript is a common task that can be achieved using various methods. By understanding the importance of unique values in programming and exploring these methods, you can improve the effectiveness and efficiency of your code.
Try what you have learned in the interactive code editor below.
Have fun coding!